Features
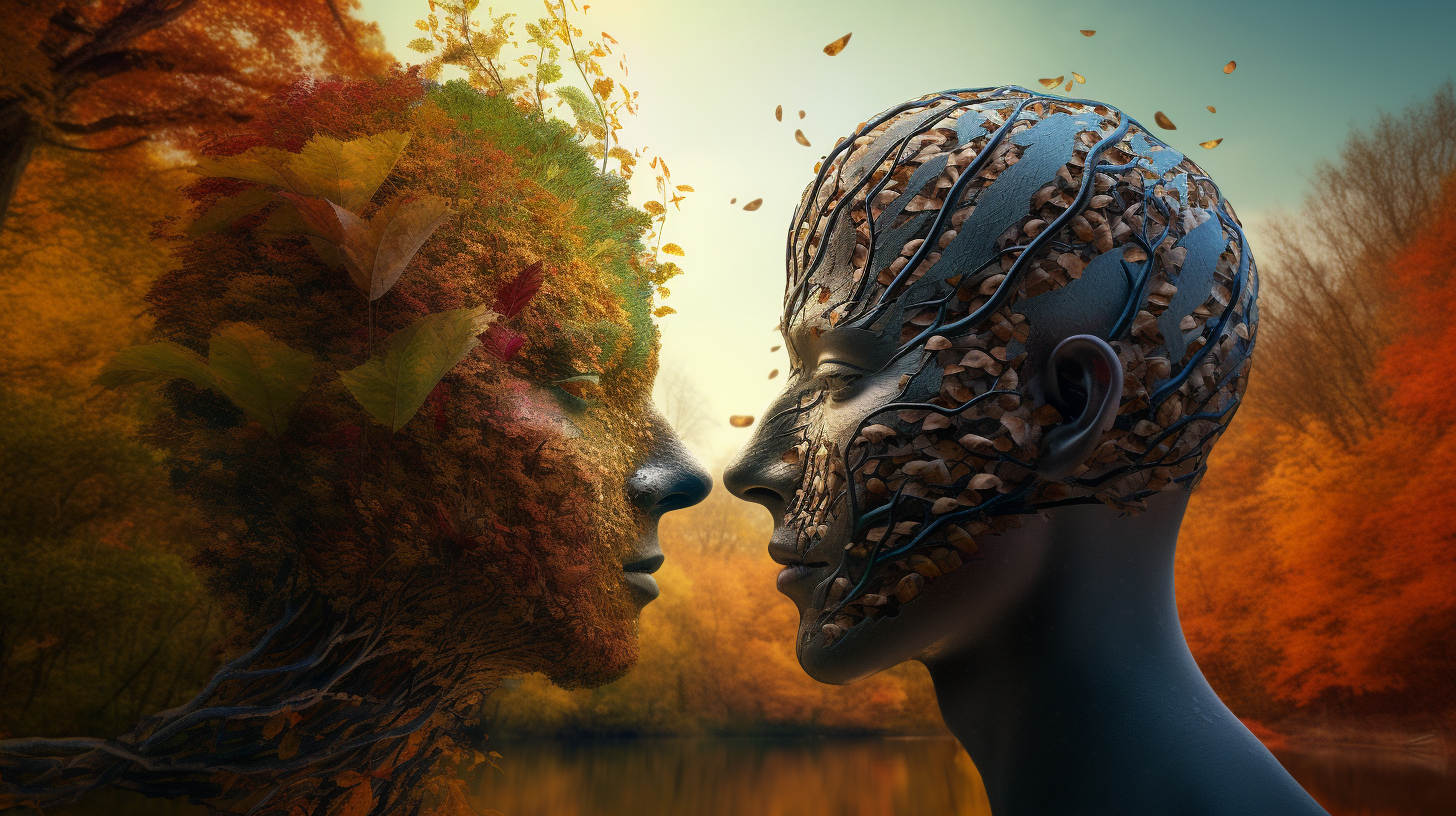
Architecture
6,619 views
Technical designs and discussions about cloud-based systems.
AI,ChatGPT,Deforum,Midjourney
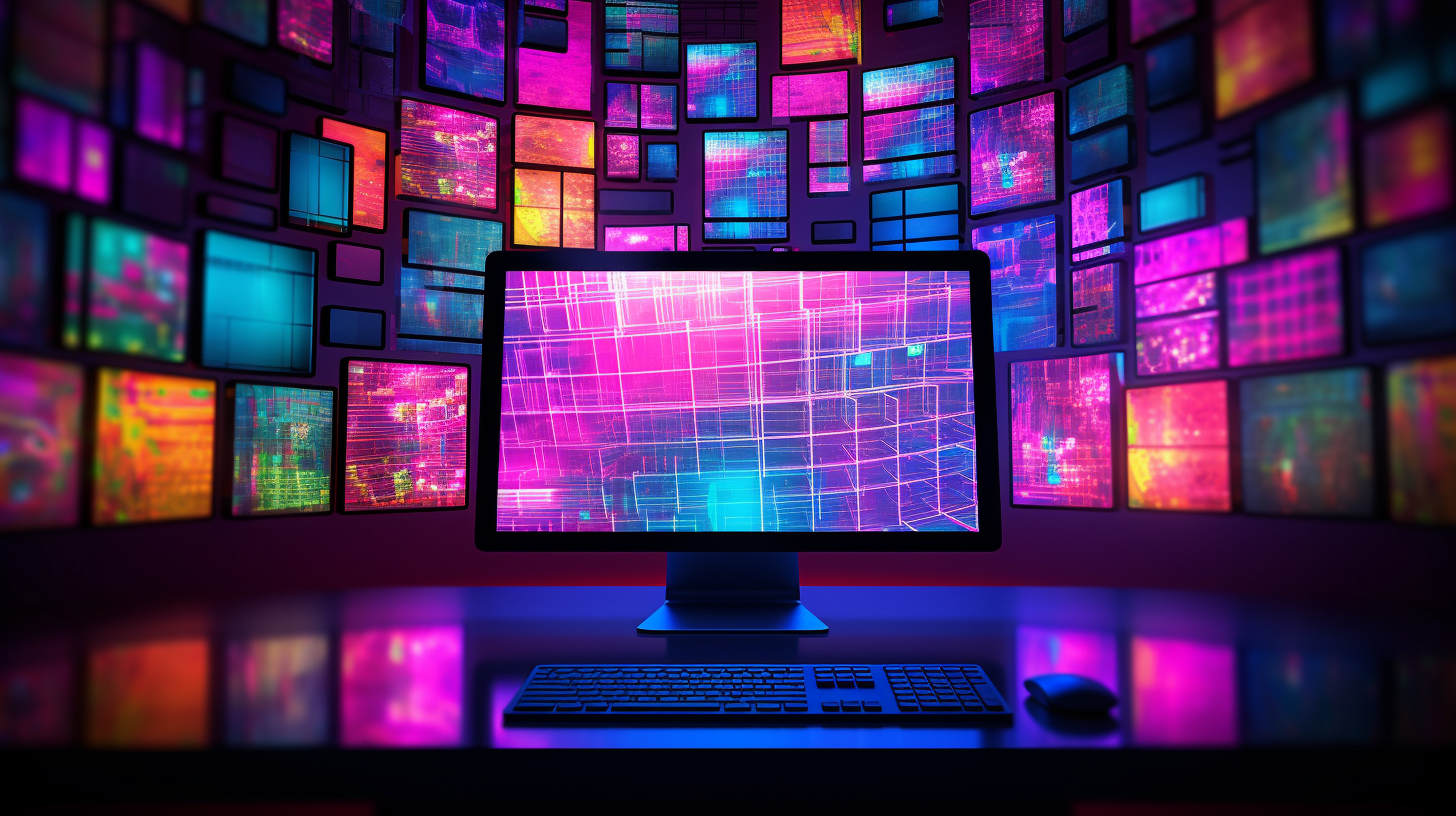
Software
7,846 views
Articles and diagrams on writing code and solving technical problems.
Architecture,AWS,Code,Diagram
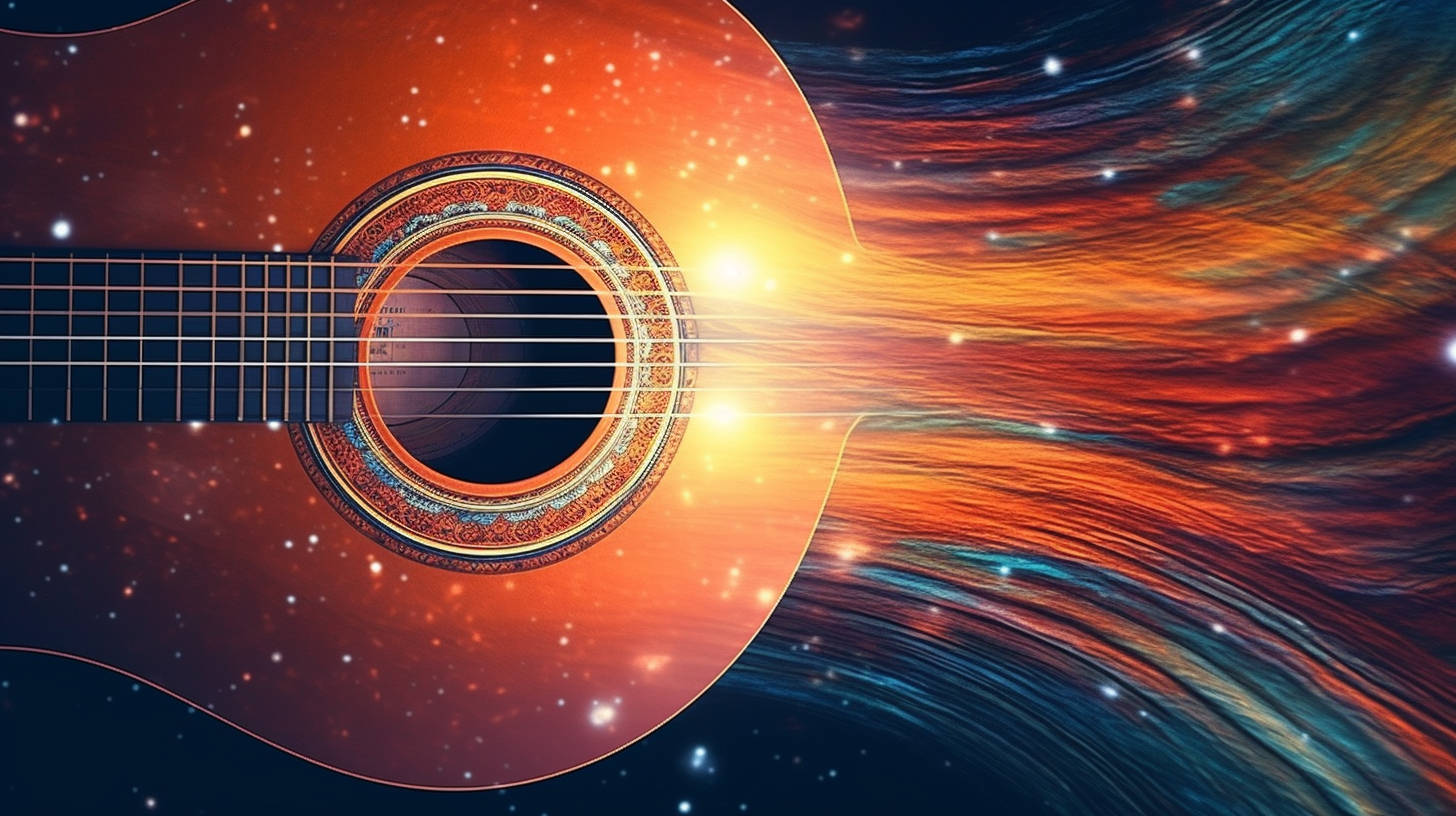
Management
6,284 views
Running a company and working with teams of engineers to deliver quickly.
Music,Playlist
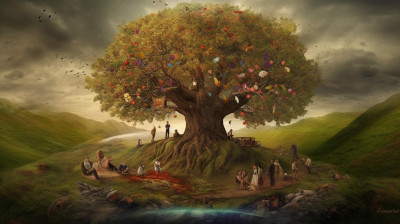
Creativity
5,792 views
Videos and music, graphic design, original and AI-generated art.
Deforum,Midjourney,Original Art,Video
Architecture
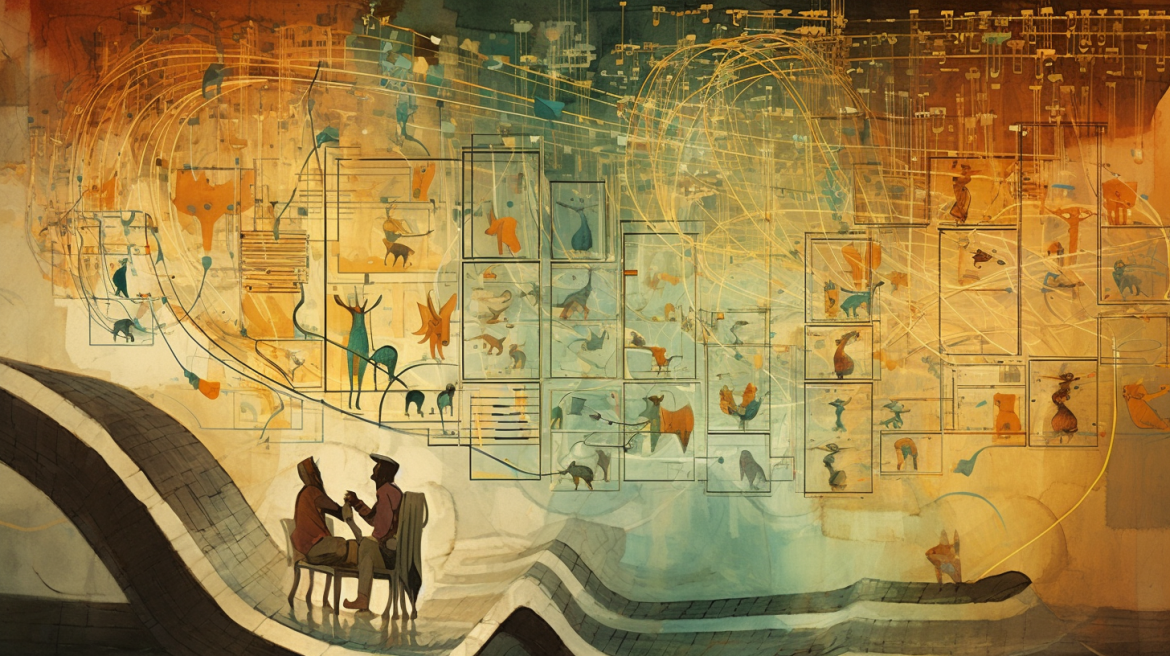
AWS AppSync Explained
9,551 views
A comprehensive dive into AWS AppSync with examples of different features and query designs. Learn how to use Lambda, DynamoDB, and HTTP data sources ...
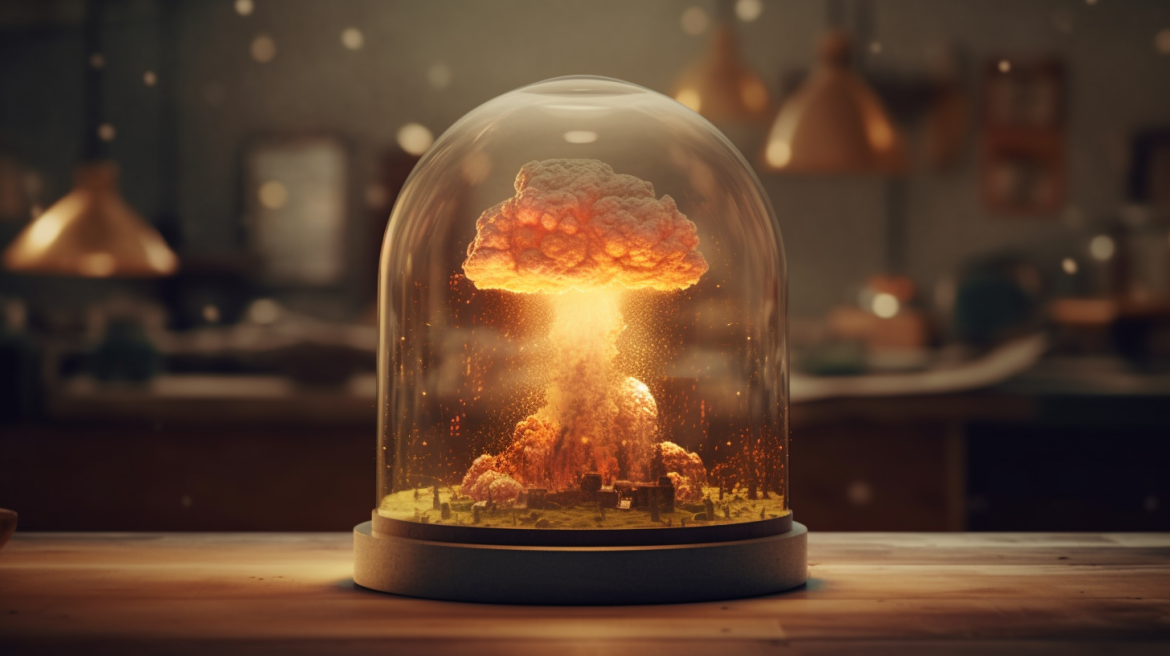
Real World Observations on CI/CD
8,596 views
Describes the transformation that happens in software development teams when CI/CD is embraced, developers own code through to production, and releasi...
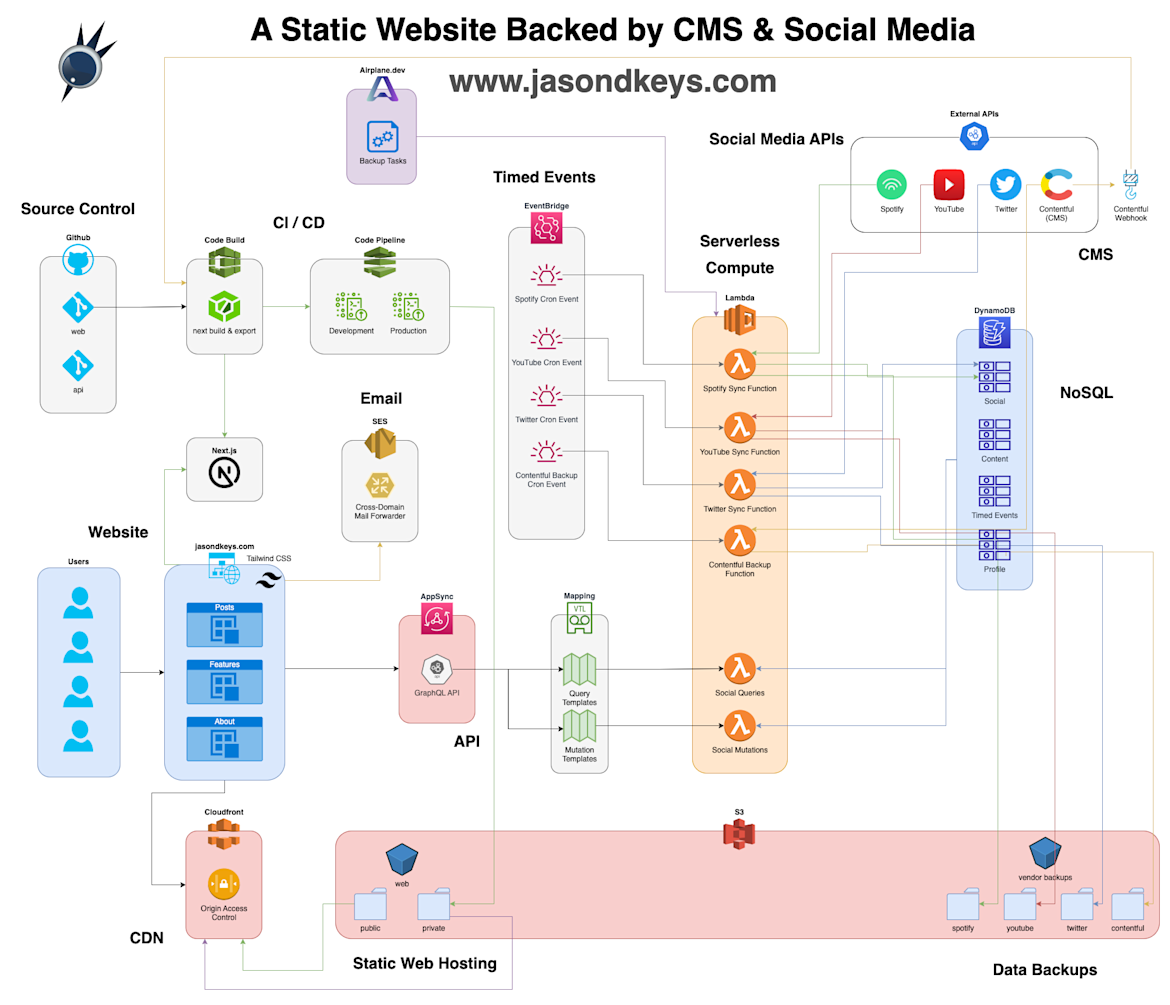
A Dynamically Static Website
9,776 views
Check out how this website works, the technology and tools that come together to make a static website come alive, and how it costs nothing to run.
Latest Videos
Johnny Cash - Big River
More Videos
Software Development
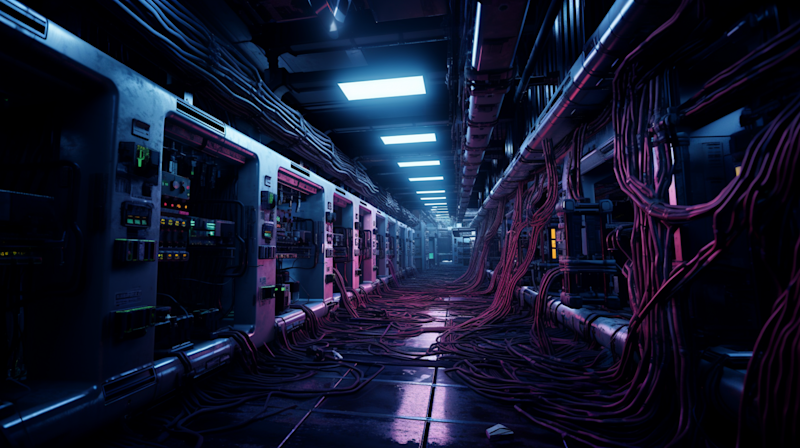
Running Stable Diffusion in AWS
9,595 views
Describes a natural evolution when creating videos with Stable Diffusion Deforum whereby self-hosting and managing compute resources with robust graph...
Code,Deforum,Stable Diffusion
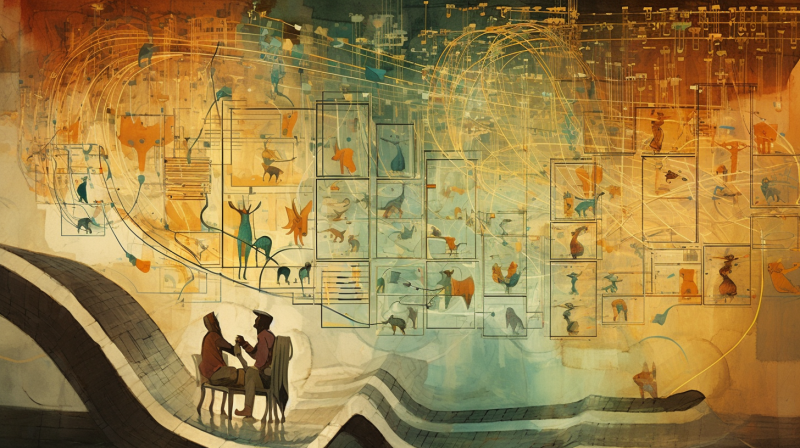
AWS AppSync Explained
9,551 views
A comprehensive dive into AWS AppSync with examples of different features and query designs. Learn how to use Lambda, DynamoDB, and HTTP data sources ...
Architecture,Code,Diagram
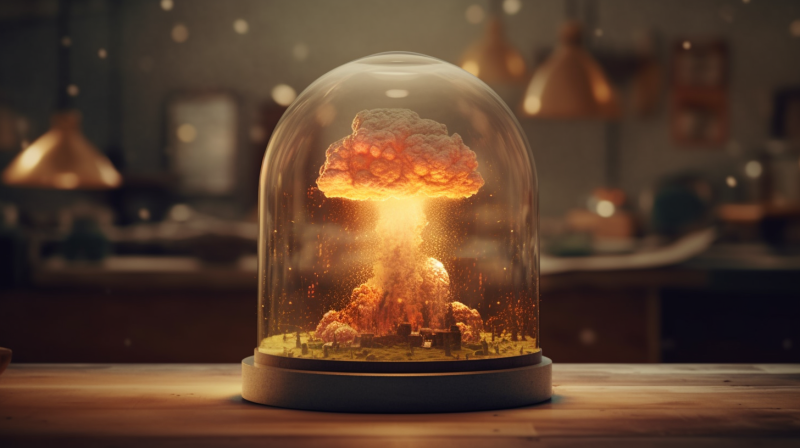
Real World Observations on CI/CD
8,596 views
Describes the transformation that happens in software development teams when CI/CD is embraced, developers own code through to production, and releasi...
Architecture,Code,Diagram

A Dynamically Static Website
9,776 views
Check out how this website works, the technology and tools that come together to make a static website come alive, and how it costs nothing to run.
Architecture,Code,Design
All Posts
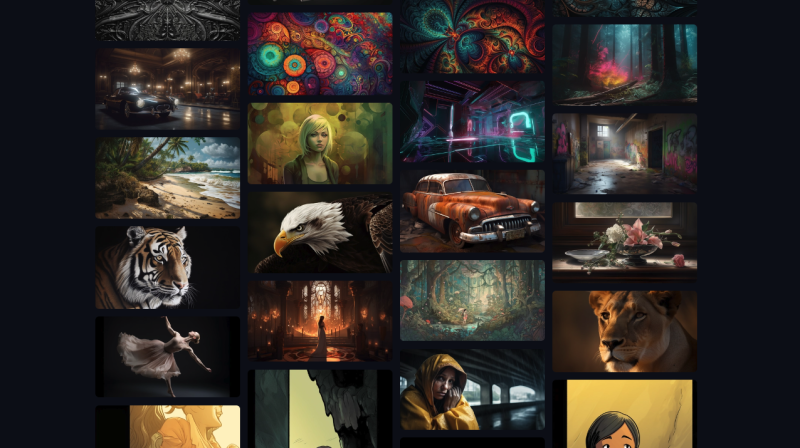
My Midjourney Profile
5,673 views
Here's a link to my Midjourney portfolio. You can check out all the images I'm creating for use on this site and my YouTube channels.
AI,Midjourney
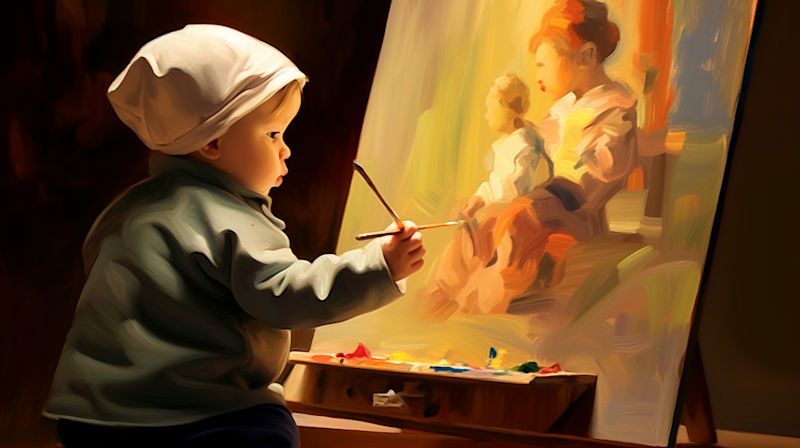
Generative AI Art Links
6,408 views
A collection of links to begin generating AI art both in the cloud for free and locally on your computer. Follow along with the links I used to go fro...
AI,Deforum,Midjourney
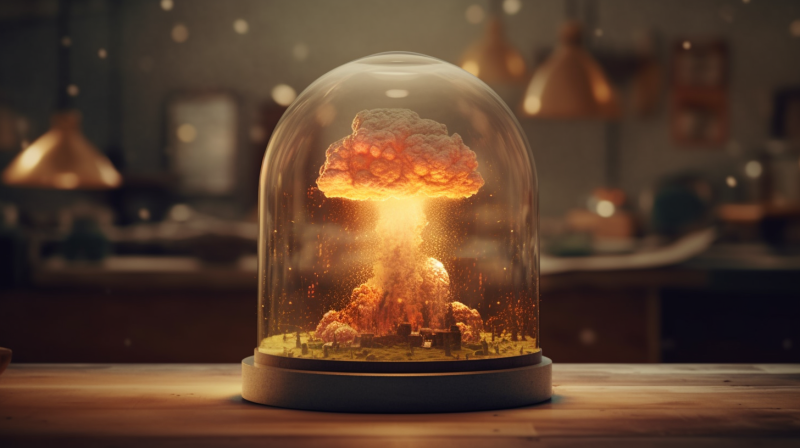
Real World Observations on CI/CD
8,596 views
Describes the transformation that happens in software development teams when CI/CD is embraced, developers own code through to production, and releasi...
Architecture,Code,Diagram
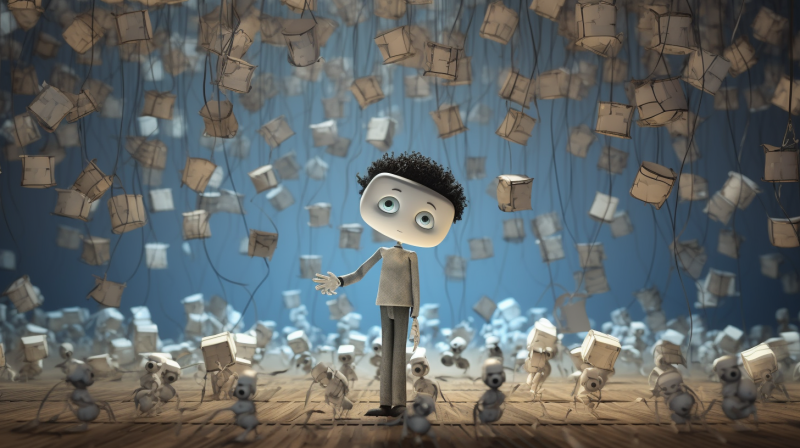
Synthesizing My Voice
7,778 views
I use ElevenLabs Speech Synthesis tool to synthesize my voice in minutes. Now I have a vocal clone I use to read my articles and say whatever I don't ...
AI,Technology
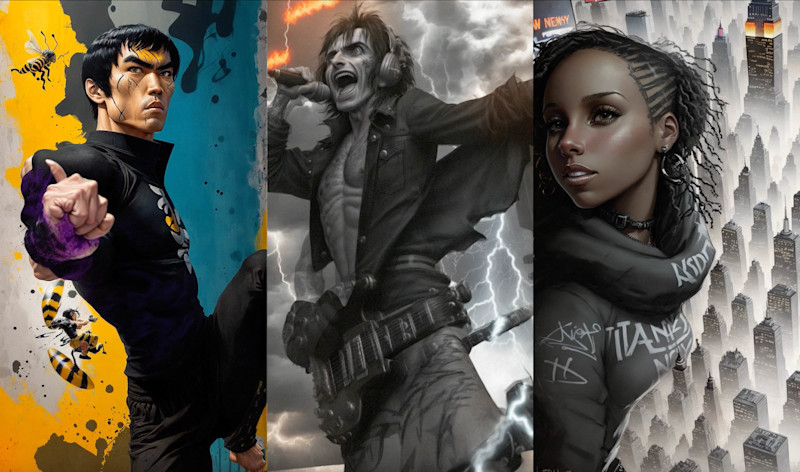
Deforum YouTube Shorts 6
8,101 views
The Wu Tang Clan, AC/DC, and Jay Z show up in 3 more YouTube shorts created with Stable Diffusion Deforum, the Rev Animated model, and 2D/3D motion ef...
AI,Deforum,Stable Diffusion
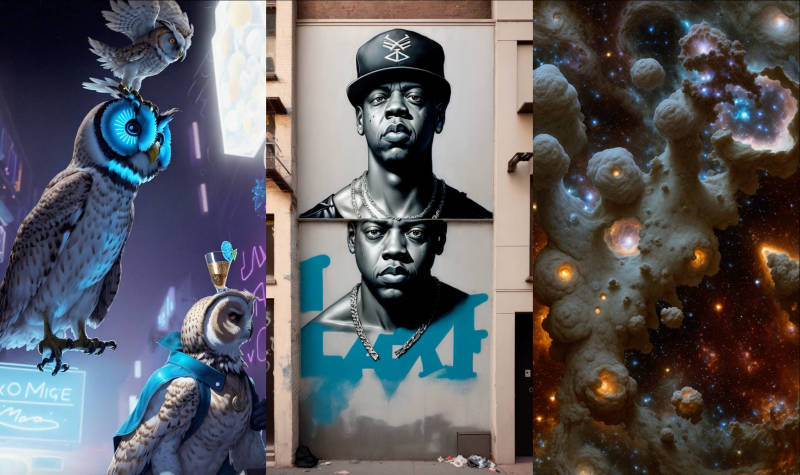
Deforum YouTube Shorts 5
7,935 views
I continue to post YouTube Shorts using Stable Diffusion Deforum. Here I set them against Pink Floyd, Drake, Jay Z and Kanye West. The channel is gain...
AI,Deforum,Stable Diffusion
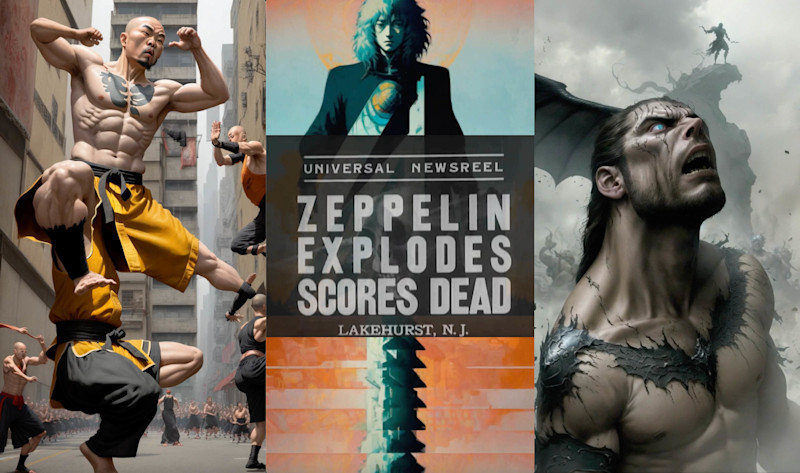
Deforum YouTube Shorts 4
9,499 views
Today I created 3 more videos with Deforum in RunDiffusion. I used the RevAnimated model, DPM++ SDE Karras Sampler and 3D motion effects to once again...
AI,ChatGPT,Deforum
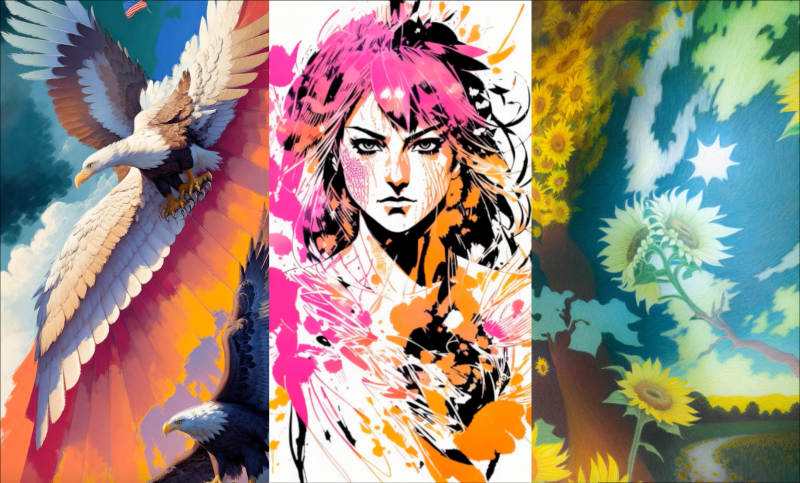
Deforum YouTube Shorts 3
5,963 views
More videos created with Deforum, Stable Diffusion 1.5 and RevAnimated 1.2.2 models, Euler A and DPM++ SDE Karras samplers, and both 2D and 3D oscilla...
AI,ChatGPT,Deforum
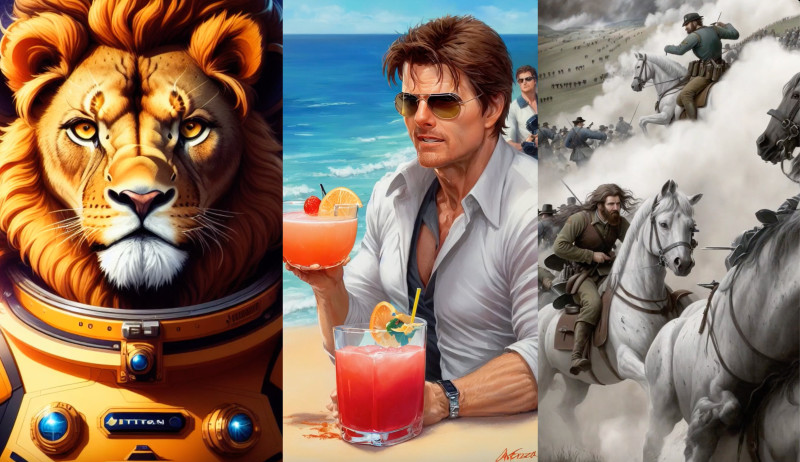
Deforum YouTube Shorts 1
7,079 views
I use Stable Diffusion Deforum, Run Diffusion, and ChatGPT to generate YouTube shorts with incredible visuals and licensed music.
AI,ChatGPT,Deforum
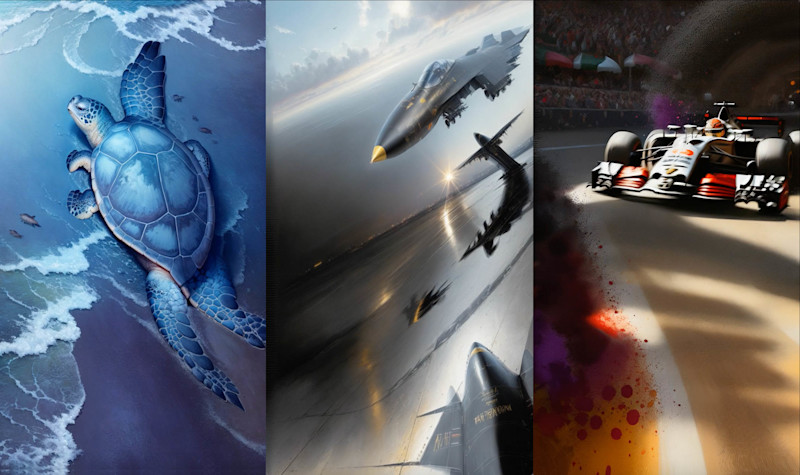
Deforum YouTube Shorts 2
5,374 views
I continue using Stable Diffusion Deforum with the RevAnimated model. Here are a few videos of ocean animals, Valkyries, and Formula 1.
AI,ChatGPT,Deforum
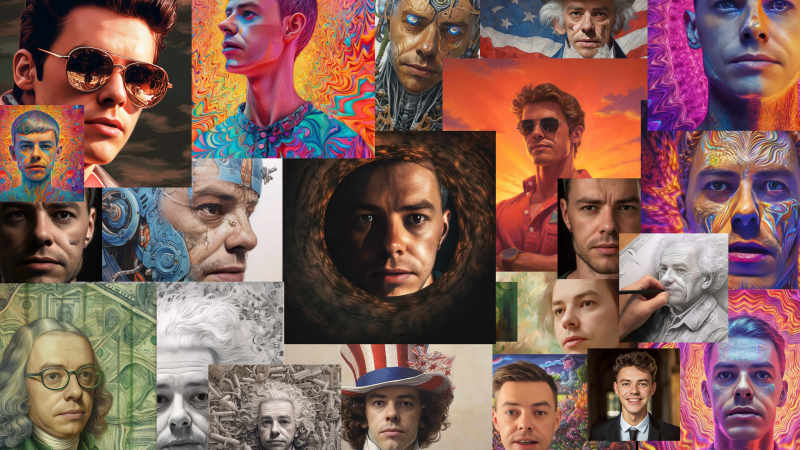
Swapping Faces in Midjourney
7,236 views
I use the InsightFace analysis library bot in Midjourney to add my face to any image I generate.
AI,Midjourney,Stable Diffusion
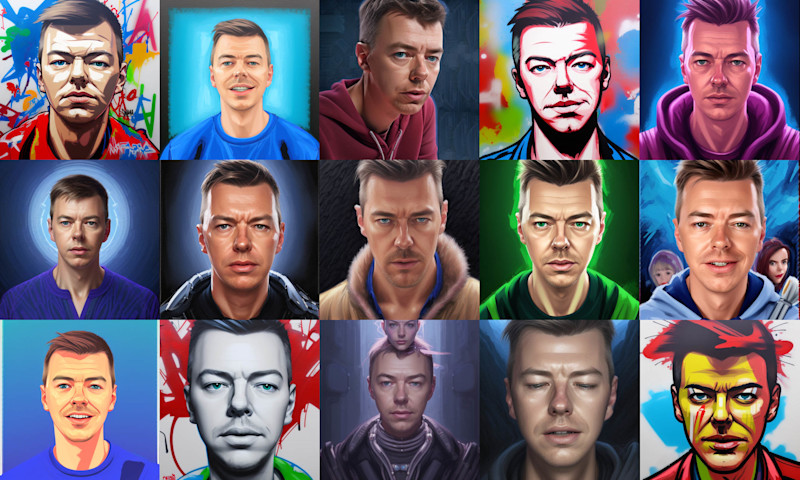
Running Stable Diffusion Locally
6,136 views
Today I installed Stable Diffusion locally, launched the web UI, and used a model I trained previously on my own face to generate pictures of me in di...
AI,Stable Diffusion

Stably Diffusing Myself
5,867 views
I use Dreambooth, Google Colab, and Hugging Face to train Stable Diffusion on my face so I can generate pictures of myself in different worlds and sty...
AI,Stable Diffusion
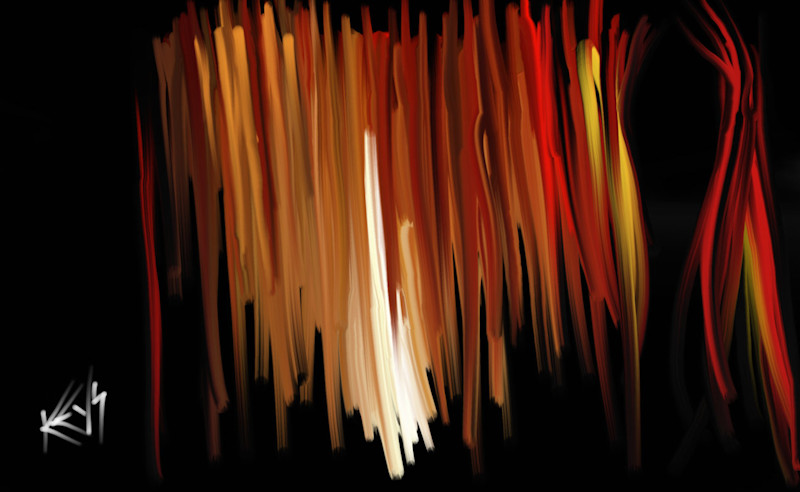
Supercars Soundtrack
9,715 views
Here is the soundtrack I created for the Supercars video I posted to my children's YouTube channel. I did everything in single takes. So I just came u...
Music,Original
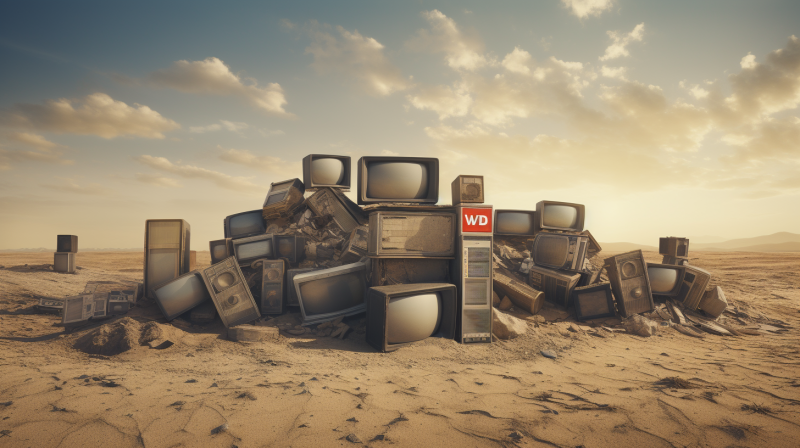
YouTube Shorts with Licensed Music
6,058 views
Here are some YouTube Shorts I've created the past few weeks that utilize licensed, popular music available when uploading a Short from your phone.
Video
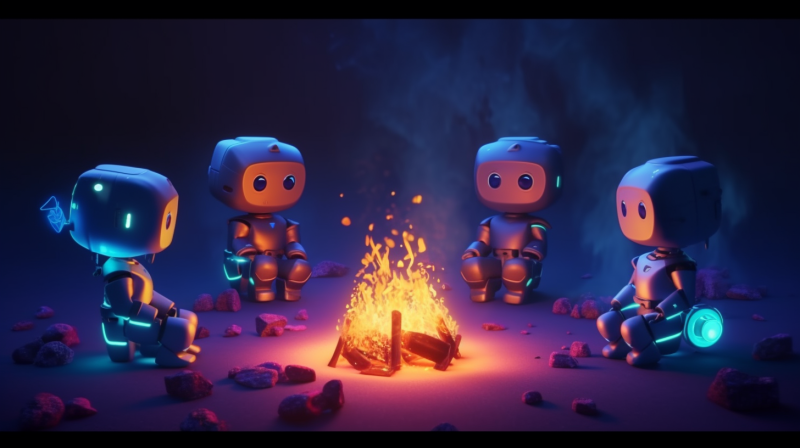
Kids Talk AI YouTube Channel
6,244 views
I created a YouTube channel on artificial intelligence that includes facts, fears and thoughts on the future presented by an AI child's voice. It is i...
AI,Video
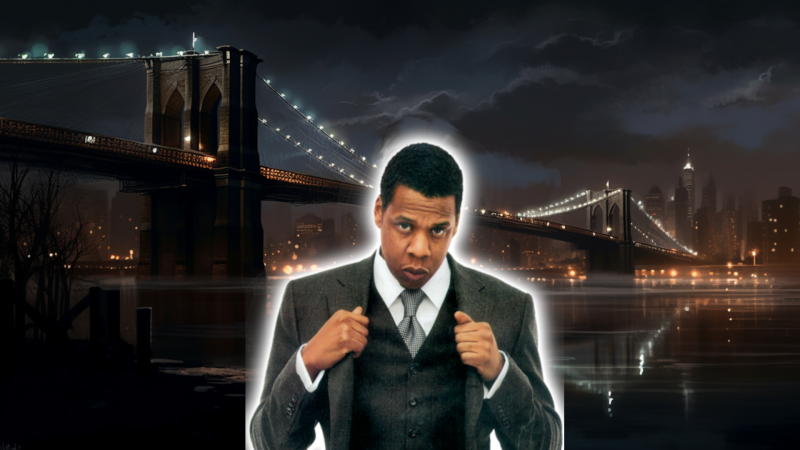
Presenting Jay-Z with Pictory
6,273 views
I use ChatGPT and Pictory to create a video with quotes and lyrics from Jay Z. I'm able to finish the 4-minute video in 40 minutes. Check out the vide...
AI,ChatGPT,Video

Some Wisdom from Jiddu Krishnamurti
7,738 views
I've started a YouTube channel called Rise and Shine Quotes that aims to resurrect the words of forgotten thinkers and present them in digestible vide...
Video
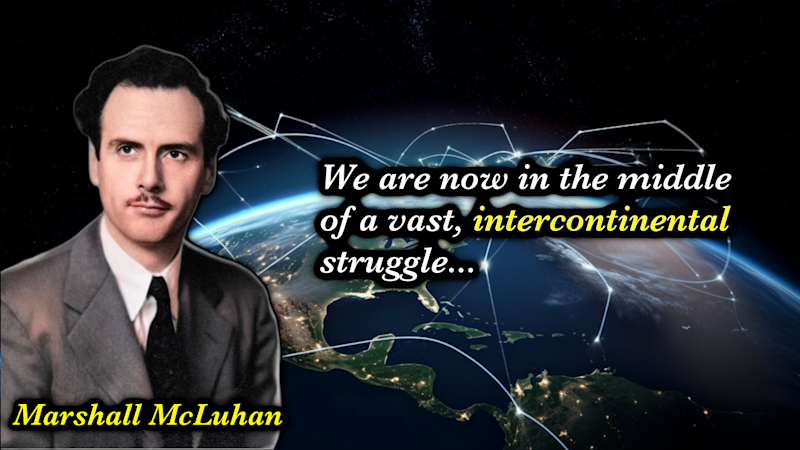
Using Pictory to Present McLuhan
8,300 views
I use Pictory AI to create a four-minute video in under an hour. Now I can focus on testing different niches for my videos more quickly with the help ...
AI,ChatGPT,Video
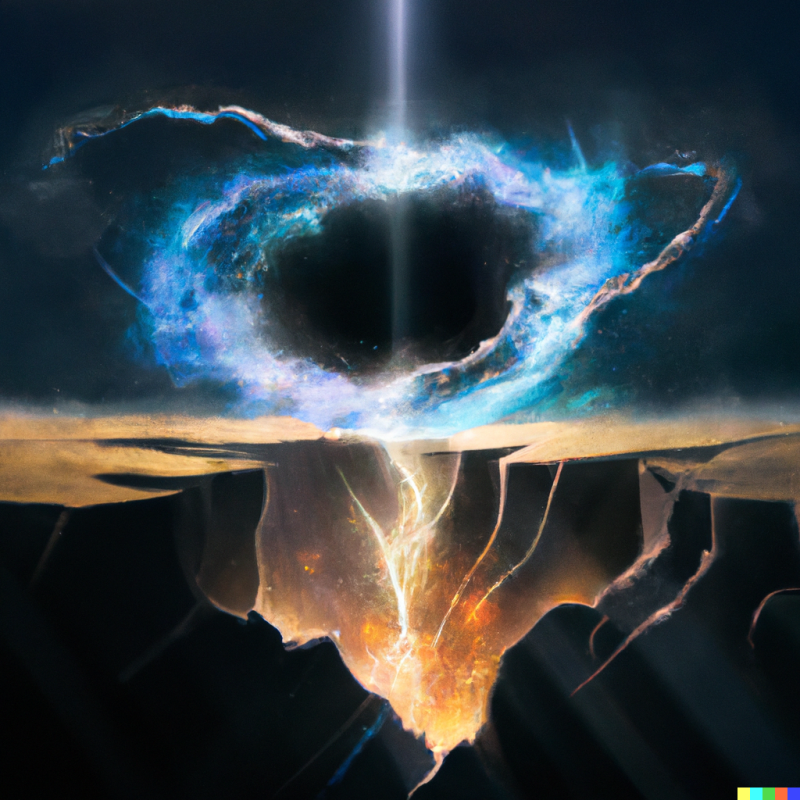
Sci-Fi Art with DALL-E
7,095 views
Here are some sci-fi themed outputs from DALL-E. With the right prompt, it can produce some very interesting images. And until Midjourney is available...
DALLE
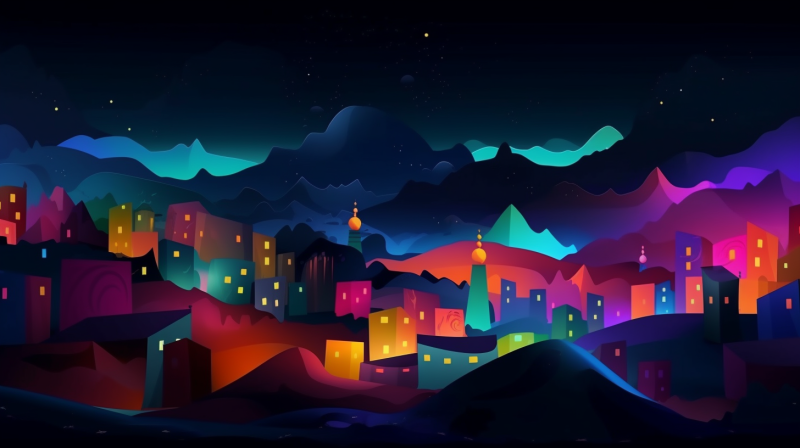
Midjourney: Banners and Landscapes
9,812 views
I used Midjourney to create background images, YouTube banners, and landscapes for videos, channels and games. I'm learning about animation and creati...
Midjourney
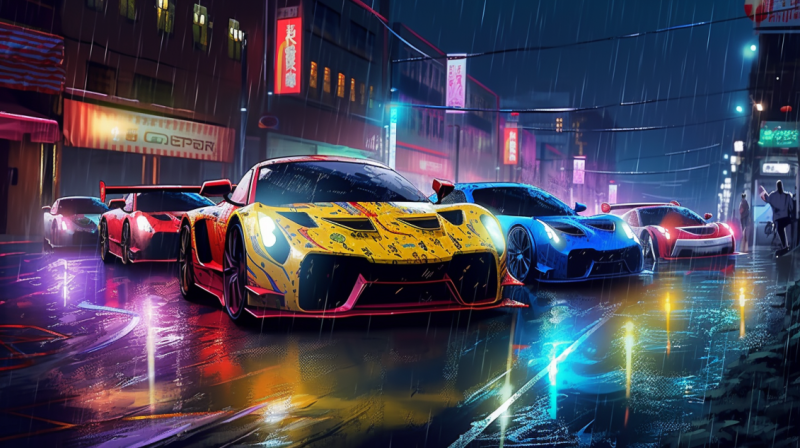
Supercars Video
5,636 views
I used Midjourney to create images of Supercars in an anime vaporwave style for a children's video. I wanted to see the cars at night in the rain stre...
Midjourney,Video

ABCs Video
9,193 views
I used Midjourney to create some interesting mashups of letter and word for a children's video on the ABCs. Check out the video and some of the pictur...
Midjourney,Video

Animals In Clothes Video
8,726 views
Here are some of the raw DALL-E images that became assets in my video "Animals In Clothes". I was trying to generate old-looking paintings in the styl...
DALLE,Video
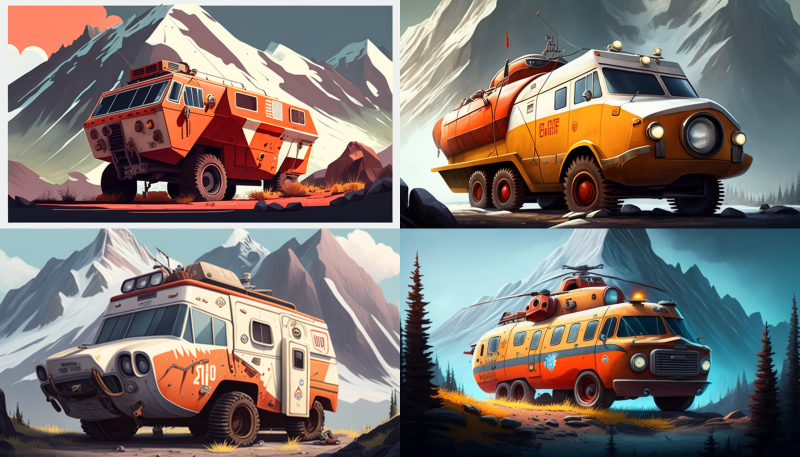
Emergency Vehicles Video
9,798 views
Here is a video I made recently and the raw Midjourney images I used as assets. I was going for a Pixar Cars style because my son is currently obsesse...
Midjourney,Video

A Dynamically Static Website
9,776 views
Check out how this website works, the technology and tools that come together to make a static website come alive, and how it costs nothing to run.
Architecture,Code,Design
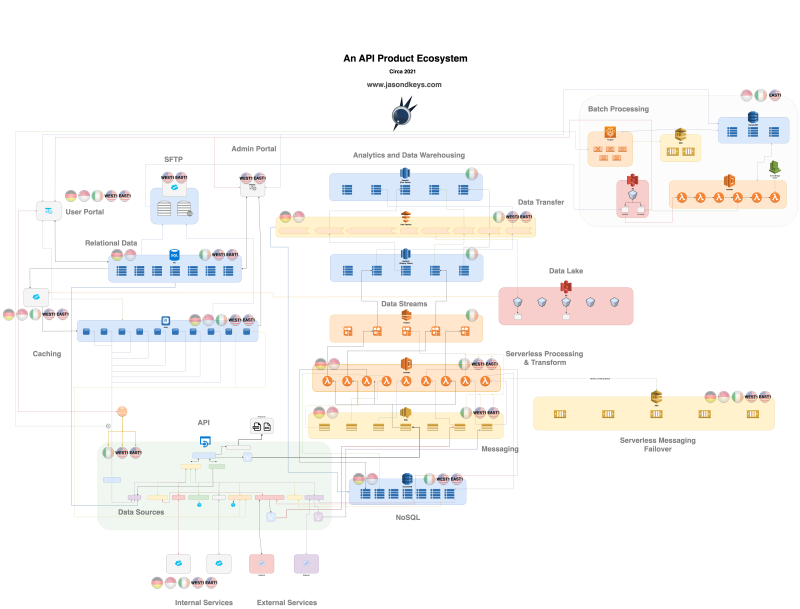
The Architecture of a Robust API
7,165 views
A technical picture that shows how a large API product may look in the AWS ecosystem, its web of connections and the high-level concerns in each area ...
Architecture,Code,Design
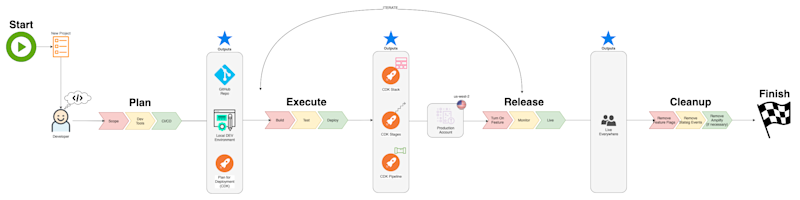
Code Releases and Competitive Advantage
5,636 views
Explores the changes CI/CD brings to company with an economic lens, seeing how the frequency of code releases represents a competitive advantage for t...
Code
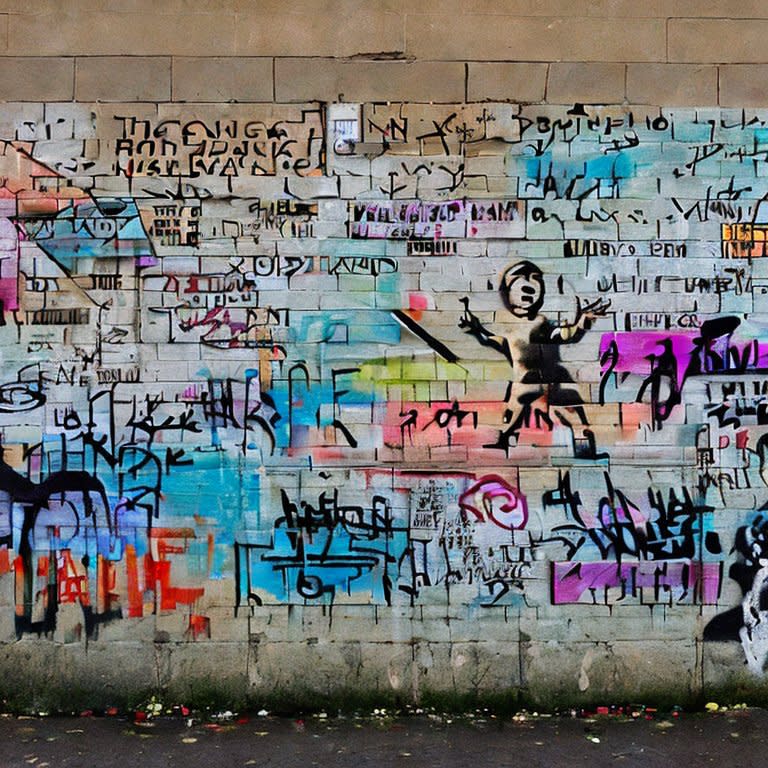
Outsourcing Web Development to AI
7,094 views
ChatGPT plays the role of teacher and junior developer to me as I build user interfaces and regain my sanity.
AI,ChatGPT,Code

Learning the Developer Mindset
6,744 views
Discusses how software developers must continually reinvent themselves and learn new tools to stay relevant.
Code,Design
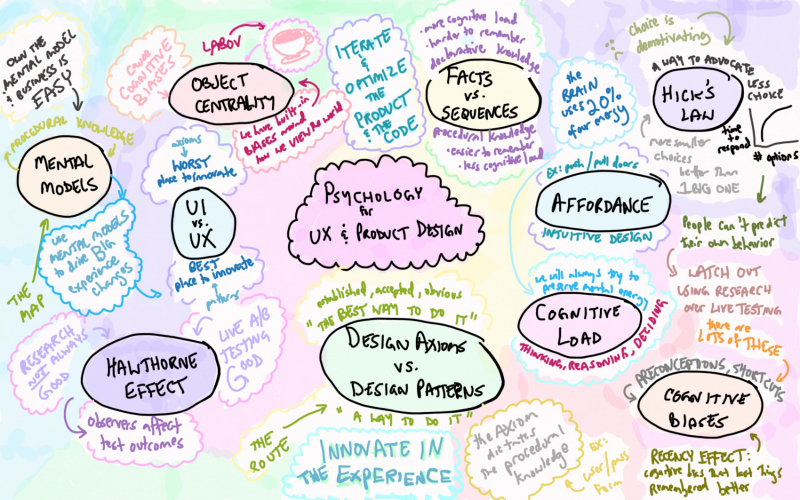
Psychology for UX & Product Design
5,747 views
Summary notes of an online workshop called Psychology for UX and Product Design where I learned about cognitive load, affordance and Hick's Law.
Design,Drawing,UI
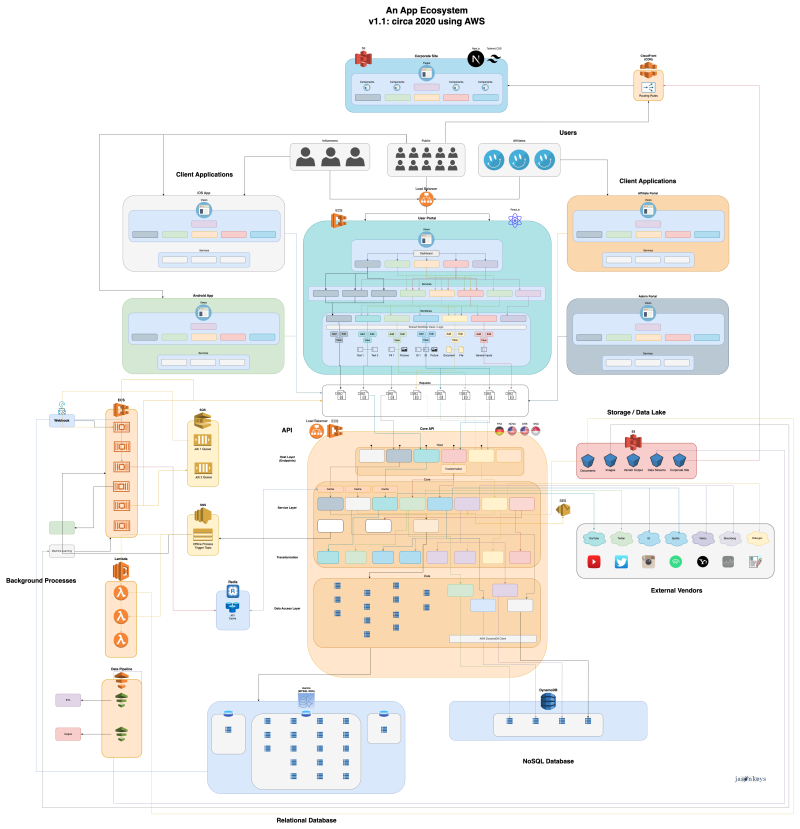
How I Make Technical Diagrams
9,148 views
Discusses how I collaborate on requirements, sketch out solutions, and produce beautiful technical diagrams.
Architecture,Code,Design
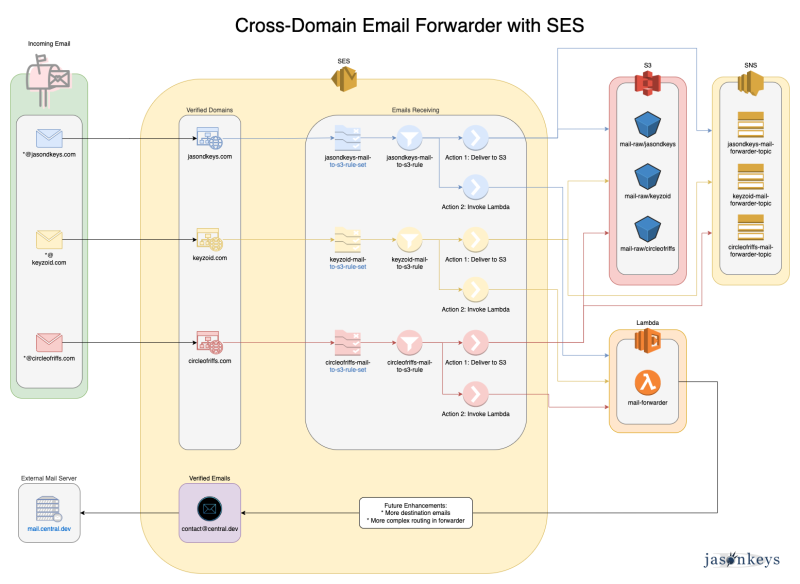
Implementing a Cross-Domain Email Forwarder with SES
6,851 views
Using AWS SES to forward disposable cross-domain email addresses to a shared third-party mail server.
Architecture,Code,Design
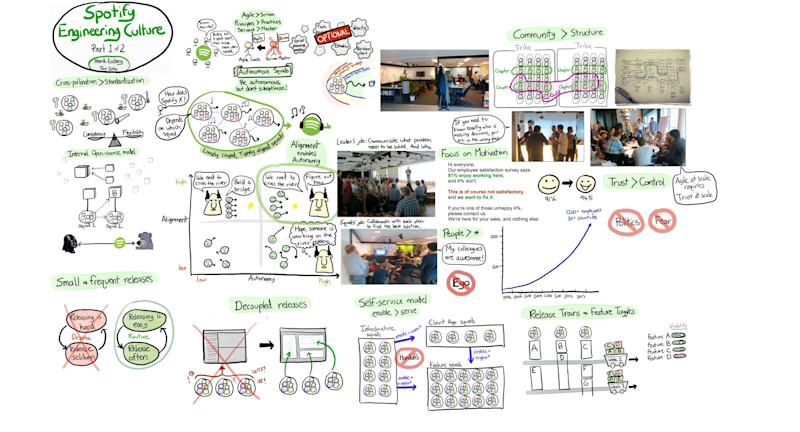
A Startup Story: How Dev Teams Take Control
7,461 views
How an engineering team takes control in a startup by controlling the speed and integrity of the release process.
Architecture,Code
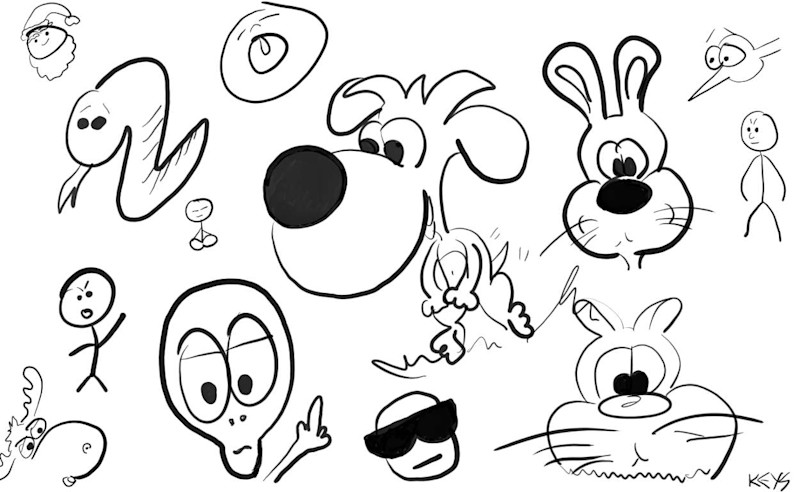
Cartoon Poses and Cameos
9,504 views
I've always loved drawing cartoons. My school notebooks growing up had every inch covered with them. I still draw them all the time. Sometimes on a ta...
Drawing,Original
Quotes
The age of writing has passed. We must invent a new metaphor, restructure our thoughts and feelings.